Preparing for Data Science Interview
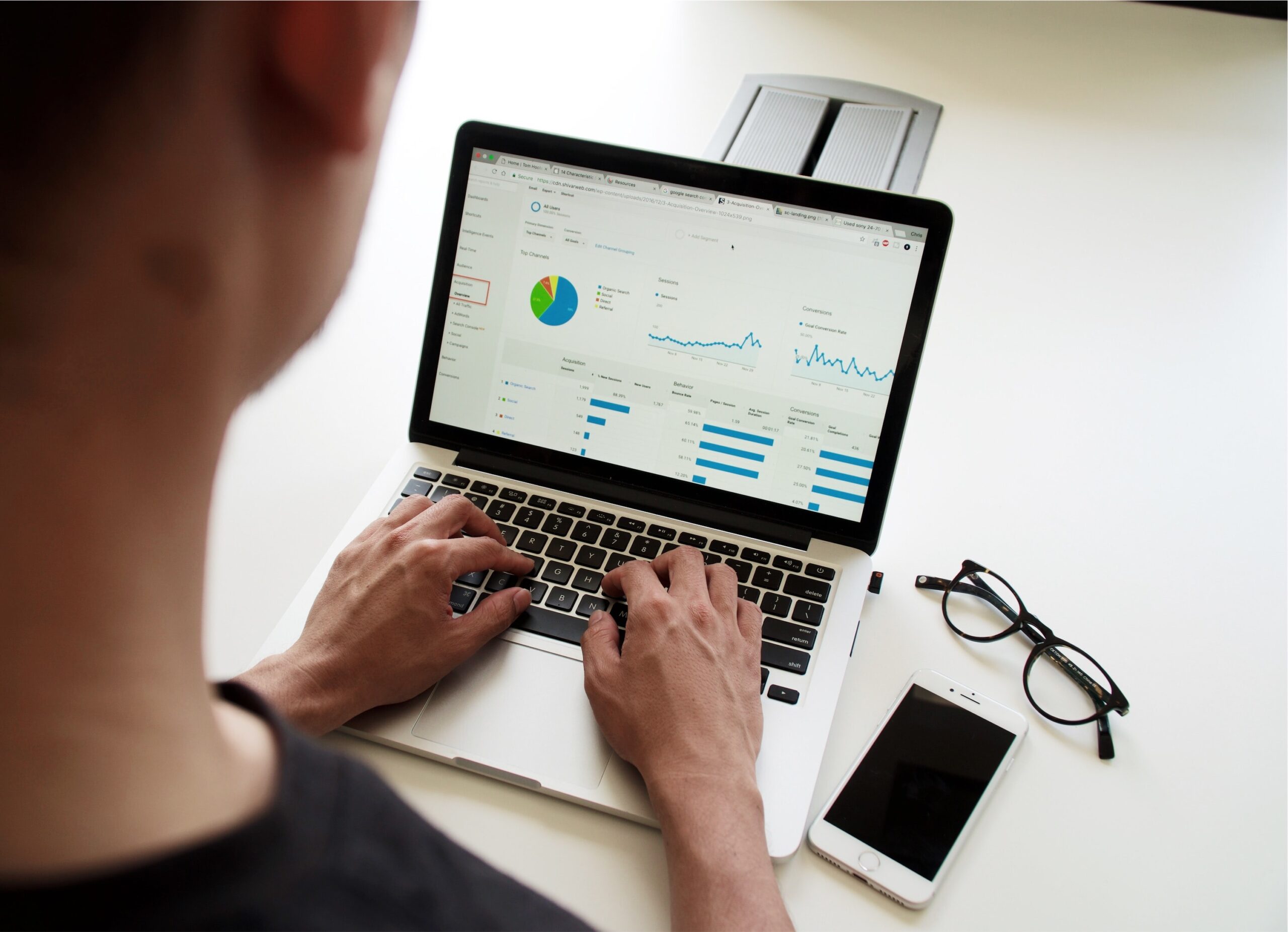
While preparing for data science/machine learning interviews, I realized there was a lot of knowledge to cover for the different rounds of the interview process. In order not to be overwhelmed with information overload, I decided to put together an article on the major focus points.
My study notes were divided into different focus areas:
- Data analysis implementation
- Machine learning and deep learning algorithms
- Building and evaluating models
- Machine Learning (ML) theory
- Programming
- Statistics and probability
- Natural Language Processing (NLP)
- Recommendation Systems
- Project-based questions
- Behavioral questions
- Questions to ask your interviewer
- Questions to ask your recruiter
Data analysis implementation: Here, the focus was on syntaxes, methods, and processes for wrangling data.
- Exploratory Data Analysis (EDA):
- Using methods such as df.head(), df.shape, and df.describe for EDA of a data frame.
- Other important analyses are getting unique values in a column or the count of unique values, grouping by one or more columns, and getting aggregates.
- Handling missing values:
- Either using df.dropna(axis=0) to drop rows with missing values or df.fillna() to fill in values. You could either fill in with the mean or median of values in that column.
- Filling missing values with the mean does not work well with a diverse dataset/column that is skewed and has outliers. In this case, the median is more appropriate.
- If data was collected in some sort of other, bfill might also be a good method to use. Only fill missing data where data was not recorded, not because it does not exist. That should be kept as NaN.
- Data cleaning:
- Pandas methods for dropping columns, renaming columns, changing the data types of columns, and resetting the index.
- Subsetting a data frame based on the specific numbers, column names, or condition(s), how to merge, join, or concatenate data frames on either row or column axis.
Machine learning and deep learning algorithms
You should be able to provide an in-depth explanation and give reasons for whatever answers you give to most questions. I’ll be sharing examples of areas you should consider focusing on and some questions you should think about.
- Machine learning algorithms:
- Neural networks (NN):
- Why do you scale the features?
- What is batch normalization?
- How do you choose layers to add?
- Support Vector Machines:
- What are support vectors?
- What are kernel functions and the types of kernel functions?
- Linear and logistic regression:
- Difference between linear and logistic regression.
- Handling bias in these algorithms.
- What are solvers for logistic regression?
- Naïve Bayes:
- Why is it naïve?
- When does it perform well?
- Neural networks (NN):
- Compare machine learning algorithms:
- Classification vs regression algorithms.
- Supervised vs unsupervised algorithms.
- How do different factors affect these algorithms, e.g. decision boundaries, training data size, and speed of training?
- Different hyper-parameters for these algorithms.
- When to use which algorithm, and why (give different use cases and why they have advantages there)?
- The loss functions for different algorithms.
- Which algorithms require scaled data for the algorithms and why do they need this?
- Difference between KNN vs K-means clustering
- Cross-validation:
- What is cross-validation and why is it used in machine learning?
- When is cross-validation not necessary?
- Normalizing vs scaling vs standardization: The difference between these three terms, knowing when to use each of them and why you should choose which.
- Categorical encoding vs target encoding vs ordinal encoding:
- Definition of these encodings and differences between each.
- Use cases and examples where they could be applied.
- Generative and discriminative models:
- Explanation of these two types of models and their differences.
- Examples of machine learning algorithms under each of these categories.
- Use cases of when one of the categories works better than the other.
- Types of outputs in classification problems:
- Class outputs vs probability outputs, and how they work.
- What models give what types of outputs?
- Tree-based algorithms:
- Which algorithms are built on the tree-based model?
- What kind of data do tree-based models have an advantage on (sparse vs dense data)?
- Ensemble models:
- How they work.
- Boosting vs bagging.
- Advantages of different ensemble methods, preferred data types, and example algorithms based on each method.
- RandomForest vs GradientBoosting.
Building and evaluating models
- How to create features: Different techniques that can be used to create features from a data set.
- Build a simple model:
- Fit a model using a machine learning algorithm from scikit-learn.
- Predict the results on unseen test data.
- Tune hyperparameters: What hyperparameters are available for the selected algorithm and what do they do? Understand the different methods of tuning hyperparameters and the pros and cons of these different cross-validation techniques.
- Validating a model: Using a validation dataset.
- Feature engineering: What it is, how to do it, and why it is important.
- Confusion matrix:
- Using absolute numbers of vs percentages.
- Calculating true positive rate, false positive rate, true negative rate, and false negative rate.
- Evaluation metrics for regression vs classification models:
- Mean Absolute error (MAE), Root Mean Square Error (RMSE), R2.
- Accuracy, precision, recall.
- Negative predictive value, specificity.
- F1-score.
- ROC, AUC, ROC-AUC.
- Diversity, coverage, serendipity, novelty, etc.
- When is accuracy not a good evaluation metric for a classification problem?
- Overfitting and underfitting:
- The definitions of both theoretically and giving real-world examples.
- How to solve both problems.
- Bias-variance tradeoff and model complexity.
- Measuring feature importance.
ML Theory
- Regularization:
- Lasso regularization.
- Ridge regularization.
- Elastic net regularization.
- Comparison of L1 vs L2 regularization, the way they work, and when to use one over the other.
- Loss functions, cost functions, objective functions:
- Definitions of each of these with examples.
- The problems these functions solve.
- How do they relate to one another?
- Entropy:
- Explain entropy in machine learning.
- What machine learning algorithms use entropy and how is it applied in the algorithms?
- How do you deal with outliers?
- How do you handle imbalanced datasets in a classification model?
- How can you avoid overfitting?
Programming
- Time complexity and efficiency of Python sort and other inbuilt methods.
- Data structures and algorithms.
- Which data structures or algorithms to apply to solve a problem optimally.
- Writing code for some data structures and algorithms from scratch, and not using inbuilt methods.
- Big-O notation:
- Space and time complexity for different data structures and algorithms.
- Which to optimize for given a specific problem and limitations.
- Building queries in SQL: Basic and advanced queries focusing on syntax, efficiency, and neatness of the query.
Statistics and probability
- Statistical distributions:
- Normal, uniform, binomial, poisson, bernoulil distributions.
- Multinomial, multinoulli, uniform distributions.
- The behaviors, properties, basic calculations, and use cases of each of these distributions.
- Sampling:
- Population sampling.
- Central limit theorem.
- Random variables:
- Discrete variables.
- Continuous variables.
- Statistical analysis:
- Variance.
- Standard deviation.
- Covariance.
- Correlation.
- Regression.
- Probability theory:
- Properties of probability.
- Probability distributions (probability density function, probability mass function, cumulative density function).
- De Morgan’s law.
- Probability events:
- Independent/mutually exclusive events.
- Non-mutually exclusive events
- Disjoint events.
- How are these all different or similar (overlapping)?
- Hypothesis and A/B testing:
- Statistical significance.
- Null and alternative hypotheses, with examples.
- Type I and II errors.
- What are the p-value, statistical power, and confidence level, and how are they calculated?
- Bayes’ rule:
- Definition of Bayes’ rule.
- Formula to calculate conditional probability based on this rule.
- Application of Bayes’ rule in real-world probability calculations.
Natural Language Processing (NLP)
- Definitions:
- Vocabulary.
- Language model.
- Text pre-processing/analysis:
- Stemming.
- Lemmatization.
- Tokenization.
- Stop words.
- TF-IDF.
- Text vectorization:
- One-hot encoding
- Bag of Words
- Word embeddings/word vectors
- Sub-words.
- Model architecture:
- Recurrent Neural Networks (RNNs).
- Long Short-Term Memory (LSTM).
- Transformers (self-attention).
- Pros and cons of each of them.
Recommendation Systems
- Types of recommendation systems:
- Content-based
- Collaborative filtering
- Knowledge-based
- Hybrid recommender systems.
- Pros and cons of each, use-cases, and when they will not perform well.
- Explain the cold-start problem in collaborative filtering when it could occur and how to fix it.
- Ranking and clustering algorithms.
- Performance evaluation for recommender systems.
- What to consider and optimize for when designing a recommender system: Accuracy, relevance, speed, latency, diversity.
Project-based questions
- Explain your ML project process?
- What’s your favorite algorithm, and can you explain it in less than a minute?
- What are your favorite use cases of machine learning models?
- Specific questions about a project on your resume or portfolio: You should be able to talk about the end-to-end process, from the business needs, planning process, data collection, building the model, evaluating performance, deploying, and measuring performance in production.
Behavioral questions: It is very helpful to use the STAR (Situation, Task, Action, quantifiable Result) framework in answering these questions. Also, try to make it personal and take more responsibility for your work and contributions, by saying more of I, than we.
Here are some sample questions to consider while prepping:
- Tell me about yourself: Talk about your background. Describe your interests. Mention your experience. Explain why you’re excited about the opportunity.
- Why do you want to work with the company?
- What do you think is the most valuable data in business?
- What has been the most significant accomplishment in my career so far / biggest success?
- Where do you want to take your career? What do you want next?
- Describe the last time you had to adjust your course to reach a goal more effectively? What was the goal, how did you adjust, and what was the outcome?
- Would you prioritize speed of delivery or quality of the product?
- Talk about a project that you worked on that failed.
- Talk about a time when you didn’t think you could do something.
- Talk about a time when the people around you disagreed about something, and how you resolved it.
Questions to ask your interviewer
- Ask about the day-to-day responsibilities of the role for which you have been interviewed.
- What makes the best intern (new hire) on their team stand out / what are the key values and characteristics that they look for in an employee?
- What are the metrics on which your performance will be evaluated while working in the company / what are the expectations for this role?
- Is there anything about your experience or skills that they have reservations about? If so, let them know that you would like to address their concerns.
- What is the typical career path for someone hired for this role?
- What is their favorite part about working in this company and what is the most challenging aspect of this job?
Questions to ask your recruiter
- How many steps are in the interview process and what are the next steps currently?
- Is it clear yet what team you will be placed in?
- Is there room to switch teams or shadow people on other teams?
- Is there relocation or accommodation assistance?
- How long have they been with the company and why do they like working there?
- What did they think about the company before they joined and what do they think now?
Conclusion
I hope this is helpful to you, not as a comprehensive syllabus, but as a guide to the various topics you could study for your interviews. Data science is a very broad field, and the role differs by company. It is important to do in-depth research on the specific company, read the job description carefully, and speak to your recruiter to understand the focus areas to lean more towards.
I also understand that the job application and interview process can be daunting. If you are faced with rejections, please do not lose track of the fact that it is not necessarily a reflection of your knowledge gap, nor is it a measure of your worth as a human being, or a measure of your intelligence. Remember to give yourself grace, ask for feedback, make improvements in whatever way you can, and keep practicing and sending in those applications.
Please let me know what you think about this piece and kindly share this with anyone you think might benefit from this. I would also appreciate your suggestions on any other topic you might like me to write about in relation to job applications and getting data science roles.
Thank you for reading.